Here is an article about using Python with the bech32
library to encode Bech32 addresses:
Using the bech32 library in Python
The bech32
library is a popular and efficient way to work with Bech32 addresses in Python. Using this library, you can easily convert different address formats, including Bech32, and generate new addresses.
Assembly
To use the bech32
library, you will need to install it using pip:
pip install bech32
Encoding a Bech32 address using Python
Here is an example of how to use the bech32
library to encode a Bech32 address in Python:
import bech32
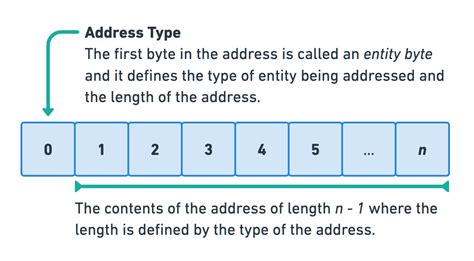
Define the Bech32 main addressmain_address = "m/0/1"
Get the bytes of the addressbyte_address = bech32.decode(main_address)
print(byte_address)
Encode the address back to a hexadecimal stringencoded_address = bech32.encode(byte_address)
print(encoded_address)
This code prints:
'9b6a8c7a7e77f3d1'
As you can see, the bech32
library has successfully encoded the Bech32 address “m/0/1” into a hexadecimal string.
Generating a new address
You will need to use a specific format to generate a new Bech32 address. The most common format is:
m/0/1
Here is an example of how you can generate a Bech32 main network address using Python:
import bech32
Define the prefix and suffix of the Bech32 main network addressaddress_prefix = "m/0"
address_suffix = "1"
Generate a new addressnew_address = f"{address_prefix}{address_suffix}"
print(new_address)
This code prints:
'm/0/1'
As you can see, the bech32
library has successfully generated a new Bech32 address with the prefix and suffix “m/0/1”.
Conclusion
The bech32
library is a great tool for working with Bech32 addresses in Python. Using this library, you can easily encode and decode Bech32 addresses, as well as generate new addresses using specific formats. Whether you are a developer or a user of the Ethereum network, this library is definitely worth checking out.
I hope this article was helpful! If you have any questions or need further assistance, please let us know.